Building Scalable Microservices with Go: A Comprehensive Guide
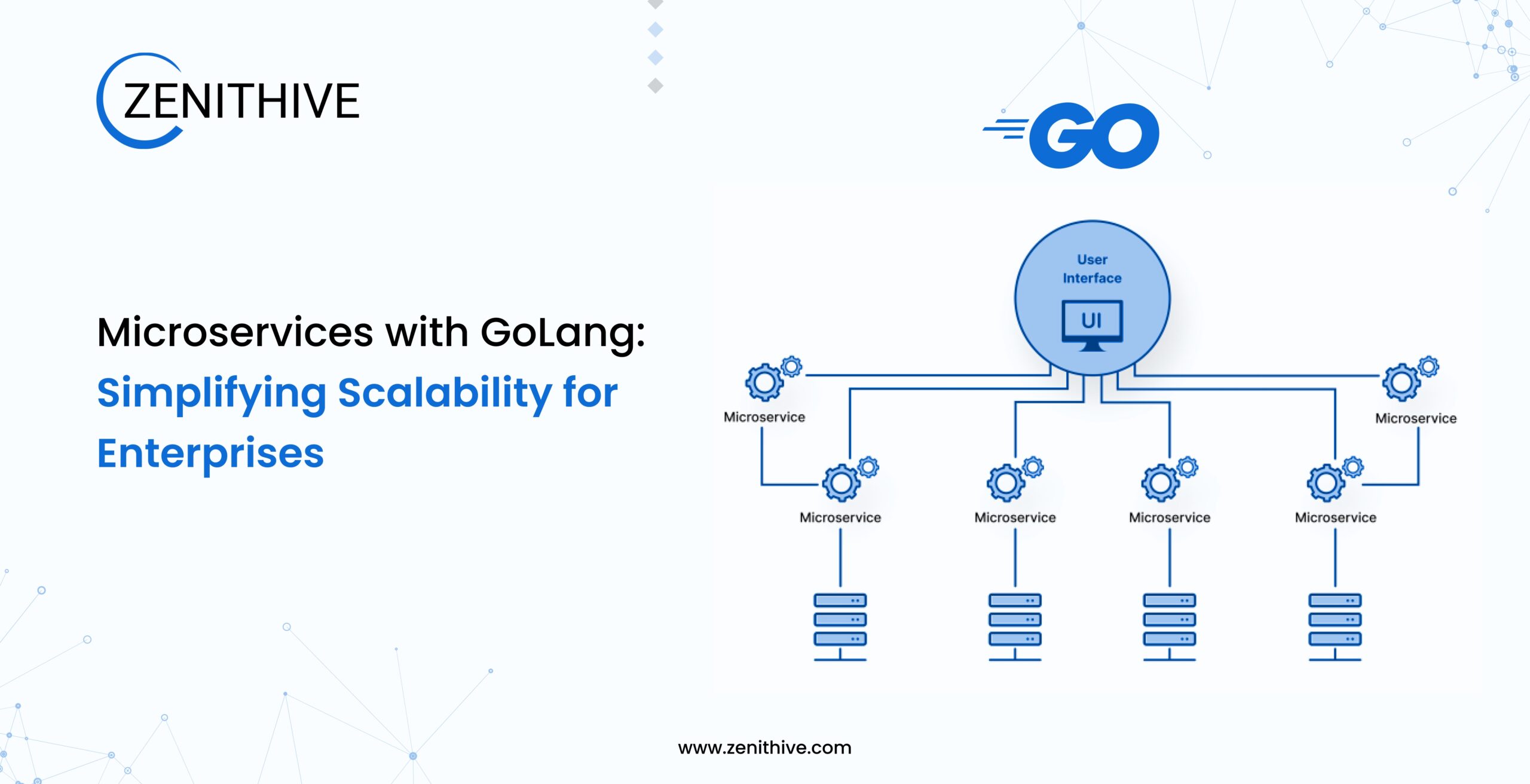
Microservices architecture has emerged as a preferred choice for building scalable, resilient, and maintainable applications. Go (Golang), with its efficiency, simplicity, and strong concurrency model, is a perfect fit for microservices development. This guide delves into the best practices, tools, and techniques for creating robust microservices using Go.
Why Choose Go for Microservices?
Go is a statically typed, compiled language designed for performance and simplicity. Here are key reasons why it’s ideal for microservices:
- Concurrency Model: Go’s goroutines and channels enable lightweight and efficient concurrent processing, making it easier to handle multiple requests simultaneously.
- High Performance: Go compiles to machine code, ensuring fast execution and low latency, which is critical for microservices.
- Scalability: Go’s built-in support for scalability helps developers design services that can handle increased loads efficiently.
- Ease of Deployment: Go produces a single binary, simplifying deployment and reducing runtime dependencies.
- Rich Ecosystem: With numerous libraries and frameworks, Go supports rapid microservices development.
Key Principles of Microservices Architecture
1. Decoupling Services
Each microservice should perform a single responsibility and communicate with others through APIs. This separation ensures that changes in one service do not impact others.
2. Scalability
Services should scale independently based on demand. Go’s inherent scalability aids in achieving this.
3. Statelessness
Microservices should avoid storing state locally, instead relying on external databases or caches to maintain consistency across instances.
4. Fault Tolerance
Design services to handle failures gracefully using techniques like retries, circuit breakers, and failover mechanisms.
5. Observability
Implement logging, monitoring, and distributed tracing to ensure you can identify and resolve issues quickly.
Setting Up a Go Microservices Project
Tools and Libraries
To get started with Go microservices, you need a robust set of tools and libraries:
- Web Framework: Use lightweight frameworks like Gin or Echo for building RESTful APIs.
- Database ORM: GORM simplifies database interactions.
- Configuration Management: Tools like viper help manage environment-specific configurations.
- Messaging: Libraries like NATS or Kafka enable asynchronous communication between services.
- Testing: Leverage GoMock and Testify for unit and integration testing.
Directory Structure
Organize your project to ensure clarity and maintainability:
project/
├── cmd/ # Entry points for the application
├── internal/ # Business logic and internal modules
├── pkg/ # Shared code across multiple services
├── api/ # API definitions and handlers
├── configs/ # Configuration files
├── migrations/ # Database migrations
└── tests/ # Test cases
Developing a Microservice
Â
Step 1: Define the Service
Start by identifying the functionality your microservice will provide. For example, a “User Service” might handle user creation, authentication, and management.
Step 2: Create API Endpoints
Design RESTful endpoints for your service. For example:
router := gin.Default()
router.POST("/users", createUserHandler)
router.GET("/users/:id", getUserHandler)
router.PUT("/users/:id", updateUserHandler)
router.DELETE("/users/:id", deleteUserHandler)
router.Run(":8080")
Step 3: Implement Business Logic
Use Go’s powerful standard library and third-party packages to implement your service logic. Ensure you adhere to the Single Responsibility Principle (SRP).
Example:
func createUserHandler(c *gin.Context) {
var user User
if err := c.ShouldBindJSON(&user); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
// Save user to database (example)
if err := db.Create(&user).Error; err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to create user"})
return
}
c.JSON(http.StatusCreated, user)
}
Step 4: Set Up Persistence
Leverage GORM or a similar ORM for database interactions. Use migrations to manage schema changes and ensure your database is version-controlled.
Example:
type User struct {
ID uint `gorm:"primaryKey"`
Name string `gorm:"size:100"`
Email string `gorm:"unique"`
Password string
}
db.AutoMigrate(&User{})
Step 5: Add Middleware
Incorporate middleware for tasks like authentication, logging, and rate limiting. The Gin framework, for example, makes it easy to add middleware components.
Example:
router.Use(gin.Logger())
router.Use(gin.Recovery())
Step 6: Integrate Messaging
For services that need to communicate asynchronously, integrate a messaging system like Kafka or RabbitMQ to publish and consume events.
Scaling and Deployment
Containerization
Use Docker to containerize your microservices. Docker images encapsulate your application and its dependencies, ensuring consistent behavior across environments.
Example Dockerfile:
FROM golang:1.20
WORKDIR /app
COPY . .
RUN go build -o main .
CMD ["./main"]
Orchestration
Use Kubernetes for container orchestration. Kubernetes automates deployment, scaling, and management of containerized applications. Key features include:
- Load Balancing: Distributes traffic across multiple instances.
- Auto-Scaling: Adjusts the number of running instances based on demand.
- Health Checks: Monitors the health of services and restarts failed instances.
Example Kubernetes Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: user-service
spec:
replicas: 3
selector:
matchLabels:
app: user-service
template:
metadata:
labels:
app: user-service
spec:
containers:
- name: user-service
image: user-service:latest
ports:
- containerPort: 8080
Service Discovery
Leverage tools like Consul or Kubernetes’ native service discovery to enable dynamic discovery of microservices within the cluster.
CI/CD Pipelines
Automate your build, test, and deployment processes using CI/CD tools like Jenkins, GitHub Actions, or GitLab CI/CD. These pipelines ensure faster and more reliable deployments.
Best Practices for Go Microservices
1. Code Quality
- Follow Go’s conventions and idiomatic practices.
- Use linters like golangci-lint to identify potential issues early.
2. Testing
- Write comprehensive unit tests for each component.
- Implement integration tests to validate interactions between services.
- Use tools like Postman for API testing.
Example Test:
func TestCreateUser(t *testing.T) {
router := setupRouter()
w := httptest.NewRecorder()
body := `{"name":"John Doe","email":"john@example.com"}`
req, _ := http.NewRequest("POST", "/users", strings.NewReader(body))
router.ServeHTTP(w, req)
assert.Equal(t, http.StatusCreated, w.Code)
}
3. Security
- Use HTTPS to encrypt communications.
- Validate all inputs to prevent injection attacks.
- Regularly update dependencies to address vulnerabilities.
4. Monitoring and Observability
- Implement structured logging using libraries like logrus or zap.
- Use monitoring tools like Prometheus and Grafana to track service health.
- Add distributed tracing with Jaeger or OpenTelemetry to analyze request flows.
5. Graceful Shutdown
Handle service termination gracefully by cleaning up resources (e.g., database connections, open files) before exiting.
Example:
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
server.Shutdown(ctx)
6. API Versioning
Maintain backward compatibility by versioning your APIs (e.g., /v1/users).
Challenges in Microservices Development
- Complexity: Managing multiple services requires robust tooling and coordination.
- Data Consistency: Achieving consistency across distributed services can be challenging.
- Network Latency: Communication between services introduces latency.
- Debugging: Tracing issues in a distributed system can be difficult without proper tooling.
Conclusion
Building scalable microservices with Go requires careful planning, the right tools, and adherence to best practices. Go’s performance, simplicity, and strong concurrency model make it an excellent choice for modern microservices architecture. By following the guidelines in this article, you can create robust, maintainable, and high-performing services that meet the demands of today’s dynamic application environments.
Looking to implement microservices in your organization or need expert guidance on Go development? Contact Zenithive today to unlock your project’s full potential and achieve seamless scalability.